Building mobile apps with React Native means you need to integrate APIs. APIs let apps talk to outside services, getting and sending data. This makes the app better for users.
Learning to use APIs in React Native can seem hard. But, with the right help, it’s easy. This guide will show you how to set up, make API calls, and deal with responses. It’s important for any app, big or small.
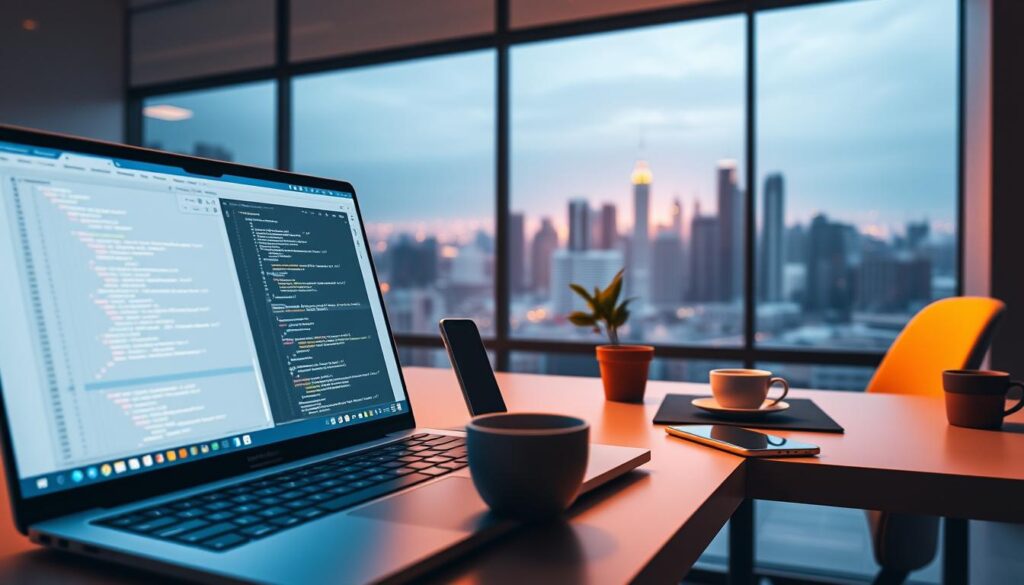
By the end of this guide, you’ll know how to use APIs in React Native. You’ll be ready to make powerful, efficient mobile apps.
Key Takeaways
- Understanding the basics of React Native API integration
- Setting up your environment for API calls in React Native
- Implementing API calls and handling responses
- Best practices for React Native API integration
- Common challenges and solutions for API calls in React Native
- Optimizing performance for API calls in React Native
Understanding API Calls in React Native
API integration in mobile development is key for building strong mobile apps. React Native, a top choice for cross-platform apps, makes it easy to use APIs. We’ll look into APIs and why they matter in mobile app creation.
An API is a set of rules that lets different software systems talk to each other. In mobile app making, APIs help get data from outside sources. This data can then be added to the app.
What is an API?
An API acts as a messenger between systems, making it easier to share data. With API integration in mobile development, apps can use services like social media or payment systems. This makes the app more useful and fun to use.
React Native’s API Integration Capabilities
React Native offers tools and libraries for easy API use in apps. There are React Native API tutorial guides and pre-made components. This makes connecting to external services quick and error-free.
Why APIs Matter in Mobile Development
In today’s fast world, APIs are crucial. Using API integration in mobile development and React Native API tutorial resources helps create better apps. These apps are more interactive, scalable, and successful, making users happy and businesses thrive.
Setting Up Your React Native Environment for API Calls
To start making API calls in React Native, you need to set up your environment. First, install Node.js and npm. These tools are key for React Native API setup. Next, configure your API integration environment. This includes setting up the API endpoint and handling API keys.
A React Native API setup needs a few key components. These are:
- Node.js: A JavaScript runtime environment that allows you to run JavaScript on the server-side.
- npm: A package manager that makes it easy to install and manage dependencies.
- API endpoint: The URL of the API you want to interact with.
- API keys: Used to authenticate and authorize API requests.
By following these steps and setting up your React Native environment correctly, you will be well on your way to making successful API calls and integrating APIs into your React Native applications.
Remember to handle your API keys securely. Keep them out of your code. This will help prevent unauthorized access to your API and keep your data safe.
Essential Methods for React Native API Calls
React Native offers several ways to make API calls. Each method has its own strengths and weaknesses. The right choice depends on your project’s needs. Let’s look at the different methods and techniques available.
Some top methods for API calls in React Native include:
- Fetch API: a built-in API for HTTP requests
- Axios: a well-liked library for HTTP requests
- XMLHttpRequest: a built-in API for HTTP requests
Using Fetch API
The Fetch API is easy and straightforward. It lets you make HTTP requests with simple syntax.
Implementing Axios
Axios is a favorite for making HTTP requests. It offers a simple API for requests and responses.
XMLHttpRequest Approach
The XMLHttpRequest approach is a built-in API for HTTP requests. It’s more detailed than Fetch API or Axios but useful in some cases.
Choosing the right API methods and techniques for your project makes development easier. It also boosts your app’s performance.
Method | Advantages | Disadvantages |
Fetch API | Simple and easy to use | Limited functionality |
Axios | Powerful and flexible | Steeper learning curve |
XMLHttpRequest | Low-level control | More complex to use |
Authentication and Authorization in API Calls
When you make API calls in React Native, it’s key to make sure your requests are secure. This is where API authentication and API authorization play a big role. They check who is making the request and let them access what they should.
To make this happen, you have a few options. These include:
- Bearer Tokens: a simple way to send a token with each request
- OAuth Implementation: a standard way to let users control who gets access to their stuff
- API Keys Management: a basic but effective method to manage who gets to use your API
It’s vital to use API authentication and API authorization to keep your APIs safe. By picking one or more of these methods, you can make sure your React Native app is safe when it talks to APIs.
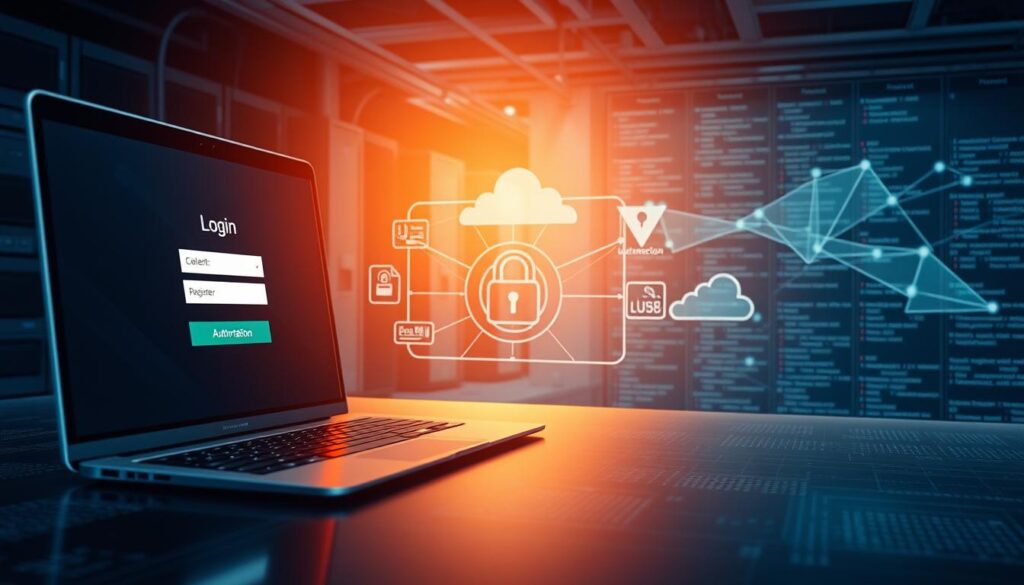
In React Native, you can use libraries and frameworks to handle API authentication and API authorization. For example, you can use the Fetch API or Axios for secure requests. By following good practices and using known libraries, you can make your API calls safer. This lets you focus on building a strong and growing app.
React Native How to Use API Call: Step-by-Step Implementation
To use API calls in React Native, you must follow several steps. First, set up your environment and install needed packages. This includes React Native and libraries like fetch or axios for API calls.
Next, create a new React Native project and go to the project directory. Then, start making API calls with fetch or axios. For instance, you can fetch data from a server with this API call example
- Import the required packages, such as fetch or axios
- Make a GET or POST request to the API endpoint
- Handle the API response and parse the data
Here’s a simple API call example using
React Native API implementation is key for mobile app development. By following these steps and using the right tools, you can fetch data from servers. For more details, check the official React Native documentation and API call example tutorial